Dungeon Raiders Proto 2 (match 3 puzzle unity)
Project 2023. 3. 17. 14:49반응형
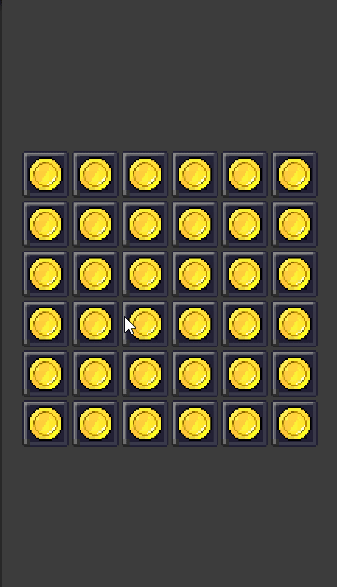
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Cell : MonoBehaviour
{
public GameObject focusGo;
public GameObject focusGo2;
public bool isSelected = false;
public int row;
public int col;
public void Init(int row, int col) {
this.row = row;
this.col = col;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.Linq;
using System;
using DG.Tweening;
public class Game : MonoBehaviour
{
public GameObject cellPrefab;
public int maxRow;
public int maxCol;
public LineRenderer line;
private Cell[,] cells;
void Start()
{
this.cells = new Cell[this.maxRow, this.maxCol];
for (int i = 0; i < maxRow; i++)
{
for (int j = 0; j < maxCol; j++)
{
var pos = new Vector2(j, -i);
var go = Instantiate(this.cellPrefab, pos, Quaternion.identity);
go.name = string.Format("({0},{1})", i, j);
var cell = go.GetComponent<Cell>();
this.cells[i, j] = cell;
cell.Init(i, j);
}
}
}
private bool isDown = false;
private List<Cell> list = new List<Cell>();
private Cell currentCell;
void Update()
{
if (Input.GetMouseButtonDown(0))
{
this.isDown = true;
}
else if (Input.GetMouseButtonUp(0))
{
this.isDown = false;
this.currentCell.focusGo.SetActive(false);
this.currentCell.focusGo2.SetActive(false);
this.currentCell = null;
//3개 이상이면 블록 제거
if (list.Count >= 3)
{
foreach (var cell in this.list)
{
this.cells[cell.row, cell.col] = null;
}
foreach (var cell in this.list)
cell.gameObject.SetActive(false);
//라인렌더러 초기화
this.line.positionCount = 0;
this.StartCoroutine(this.CoDecreaseRow());
}
else
{
Debug.Log("연결된 블록 갯수 부족");
foreach (var cell in this.list)
cell.focusGo2.SetActive(false);
//라인렌더러 초기화
this.line.positionCount = 0;
}
this.list.Clear();
}
if (this.isDown) {
var ray = Camera.main.ScreenPointToRay(Input.mousePosition);
var hit = Physics2D.Raycast(ray.origin, ray.direction);
if (hit.collider != null) {
var cell = hit.collider.GetComponent<Cell>();
if (this.currentCell != cell) {
if (this.currentCell != null) {
var prevCell = this.currentCell;
prevCell.focusGo.SetActive(false);
}
this.currentCell = cell;
if (!list.Contains(this.currentCell))
{
//처음 블록 선택
if (this.list.Count == 0)
{
Debug.Log("start");
list.Add(this.currentCell);
this.currentCell.focusGo.SetActive(true);
this.currentCell.focusGo2.SetActive(true);
}
else {
//마지막 선택된 셀과 인접해 있는가?
var dis = Vector3.Distance(list[list.Count - 1].transform.position, this.currentCell.transform.position);
Debug.Log(dis);
if (dis > 1.42f)
{
Debug.Log("인접해 있지 않음");
this.currentCell.focusGo.SetActive(true);
}
else {
this.list.Add(this.currentCell);
this.currentCell.focusGo.SetActive(true);
this.currentCell.focusGo2.SetActive(true);
//라인 렌더러
this.UpdateLine();
}
}
}
else
{
Debug.Log("이미 선택된 cell");
var idx = list.IndexOf(this.currentCell);
for (int i = idx + 1; i < list.Count; i++) {
list[i].focusGo.SetActive(false);
list[i].focusGo2.SetActive(false);
}
list.RemoveRange(idx + 1, list.Count - 1 - idx);
this.currentCell.focusGo.SetActive(true);
//라인 렌더러
this.UpdateLine();
}
}
}
}
}
private IEnumerator CoDecreaseRow() {
while (true)
{
var set = this.CalcMoveActionBlocks();
if (set.Count == 0)
{
break;
}
foreach (var x in set)
{
//Debug.LogFormat("{0},{1} -> {2},{3}", x.Item1, x.Item2, x.Item1 + 1, x.Item2);
var cell = this.cells[x.Item1, x.Item2];
this.cells[x.Item1, x.Item2] = null;
this.cells[x.Item1 + 1, x.Item2] = cell;
this.cells[x.Item1 + 1, x.Item2].row = x.Item1 + 1;
cell.transform.DOMoveY(cell.transform.position.y - 1, 0.07f);//.SetEase(Ease.InBounce);
}
yield return new WaitForSeconds(0.07f);
}
yield return null;
}
private HashSet<Tuple<int, int>> CalcMoveActionBlocks() {
HashSet<Tuple<int, int>> set = new HashSet<Tuple<int, int>>();
for (int i = this.maxRow - 1; i >= 0; i--)
{
for (int j = 0; j < this.maxCol; j++)
{
var cell = this.cells[i, j];
if (cell == null)
{
//Debug.LogErrorFormat("{0},{1}", i, j);
for (int row = i; row >= 0; row--)
{
if (this.cells[row, j] != null)
{
//Debug.LogFormat("---------->{0},{1}", row, j);
set.Add(new Tuple<int, int>(row, j));
break;
}
}
}
}
}
return set;
}
private void UpdateLine() {
this.line.positionCount = this.list.Count;
for (int i = 0; i < this.list.Count; i++)
{
var pos = this.list[i].transform.position;
pos.z = 0;
this.line.SetPosition(i, pos);
}
}
}
반응형
'Project' 카테고리의 다른 글
Dungeon Raiders Proto 4 (match 3 puzzle unity) (0) | 2023.03.17 |
---|---|
Dungeon Raiders Proto 3 (match 3 puzzle unity) (0) | 2023.03.17 |
Dungeon Raiders Proto 1 (match 3 puzzle unity) (0) | 2023.03.17 |
[게임출시] 배틀워즈 - 게임으로 즐기는 영단어 (0) | 2022.10.21 |
Flutter (플루터) 1일차 (0) | 2021.07.12 |