원격수업 C# 과제
Unity3D/C# 2023. 1. 25. 16:33인벤토리 구현
획득한 아이템들을 관리 할 수 인벤토리 시스템을 구현 해야 합니다.
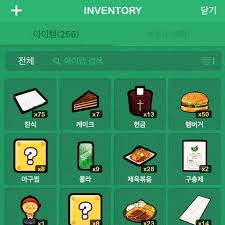
<예시 이미지>
- 아이템은 무기, 방어구와 같은 2가지 이상의 타입이 존재 합니다
- 동일한 이름의 아이템은 추가 할수 없습니다.
- 인벤토리는 최대 수량을 가지며 최대 수량이 넘으면 아이템을 추가 할수 없지만 최대 수량을 늘린수는 있습니다.
기능은 다음과 같습니다.
1. 아이템 추가
2. 아이템 삭제 (4.이름으로 검색된 아이템)
3. 인벤토리 아이템 수 확인
4. 인벤토리 최대 수량 증가
5. 아이템 이름으로 검색
6. 특정 타입으로 아이템 목록 출력
7. 모든 아이템 출력
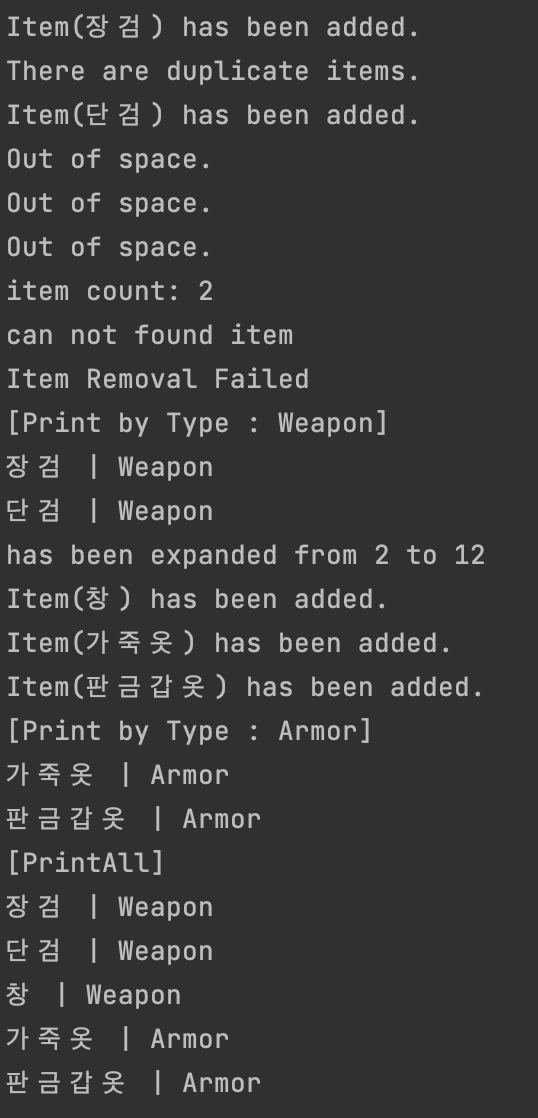
using System;
namespace Assignment01
{
class Program
{
static void Main(string[] args)
{
new App();
}
}
}
using System;
namespace Assignment01
{
public class App
{
public App()
{
Inventory inven = new Inventory(2);
inven.AddItem(new Item("장검", Item.eType.Weapon));
inven.AddItem(new Item("장검", Item.eType.Weapon));
inven.AddItem(new Item("단검", Item.eType.Weapon));
inven.AddItem(new Item("천옷", Item.eType.Armor));
inven.AddItem(new Item("천옷", Item.eType.Armor));
inven.AddItem(new Item("사슬갑옷", Item.eType.Armor));
Console.WriteLine("item count: {0}", inven.GetCount());
Item item = inven.GetItem("c");
if(item == null)
Console.WriteLine("can not found item");
else
Console.WriteLine("Item({0}) found.", item.name);
inven.RemoveItem(item);
inven.Print(Item.eType.Weapon);
inven.Expand(10);
inven.AddItem(new Item("창", Item.eType.Weapon));
inven.AddItem(new Item("가죽옷", Item.eType.Armor));
inven.AddItem(new Item("판금갑옷", Item.eType.Armor));
inven.Print(Item.eType.Armor);
inven.PrintAll();
}
}
}
namespace Assignment01
{
public class Item
{
public string name;
public eType type;
public enum eType
{
Weapon, Armor
}
public Item(string name, eType type)
{
this.name = name;
this.type = type;
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
namespace Assignment01
{
public class Inventory
{
public List<Item> items;
private int capacity;
public Inventory(int capacity)
{
this.capacity = capacity;
this.items = new List<Item>();
}
//아이템 추가
public void AddItem(Item item)
{
//Console.WriteLine("{0} {1}", this.items.Count, this.capacity);
if (this.items.Count < this.capacity)
{
var x = this.items.Find(x => x.name == item.name);
if (x != null)
{
Console.WriteLine("There are duplicate items.");
}
else
{
this.items.Add(item);
Console.WriteLine("Item({0}) has been added.", item.name);
}
}
else
{
Console.WriteLine("Out of space.");
}
}
public int GetCount()
{
return this.items.Count;
}
public Item GetItem(string name)
{
return this.items.Find(x => x.name == name);
}
public void RemoveItem(Item item)
{
if (item != null)
{
this.items.Remove(item);
Console.WriteLine("Item removed");
}
else
{
Console.WriteLine("Item Removal Failed");
}
}
public void Print(Item.eType type)
{
Console.WriteLine("[Print by Type : {0}]", type.ToString());
var iter = items.Where(x => x.type == type);
foreach (var item in iter)
{
Console.WriteLine("{0} | {1}", item.name, item.type);
}
}
public void PrintAll()
{
Console.WriteLine("[PrintAll]");
foreach (var item in items)
{
Console.WriteLine("{0} | {1}", item.name, item.type);
}
}
public void Expand(int add)
{
var temp = this.capacity;
this.capacity += add;
Console.WriteLine("has been expanded from {0} to {1}", temp, this.capacity);
}
}
}
'Unity3D > C#' 카테고리의 다른 글
유니티 C# 프로그래밍 무료 강의 (0) | 2021.09.12 |
---|---|
C# 강좌 Day-26 비동기프로그래밍 (0) | 2021.09.07 |
C# 강좌 Day-25 스레드 (0) | 2021.09.07 |
C# 강좌 Day-24 파일/JSON (0) | 2021.09.07 |
C# 강좌 Day-23 LINQ (0) | 2021.09.07 |