XR Interaction Toolkit 2.4.3 (Grab Interactable)
새 씬을 만들고 메인 카메라를 제거후 XR Origin을 생성 한다

Left/Right Controller를 선택후 XR Controller를 제외한 모든 컴포넌트를 제거 한다
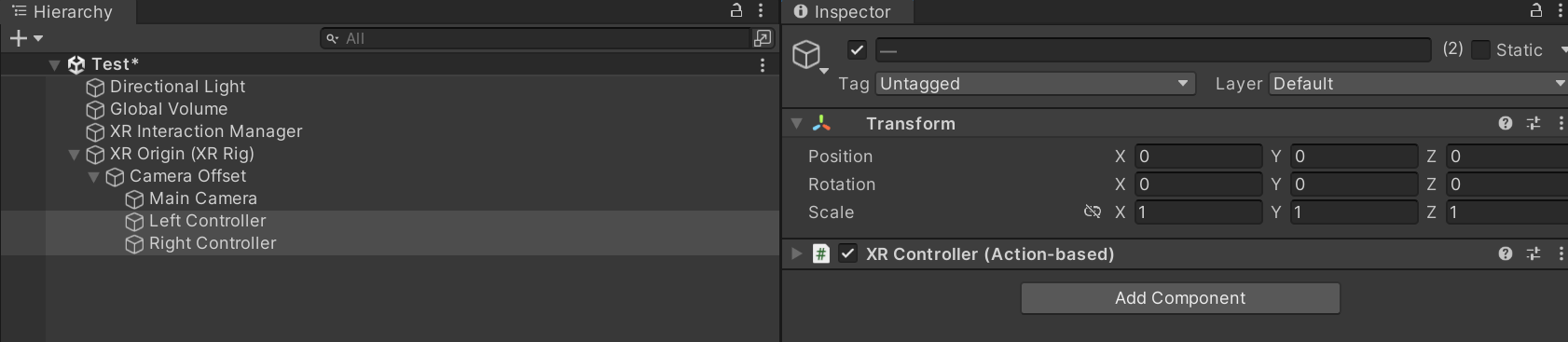
다음과 같이 구조를 만들고

핸드 모델을 Offset자식으로 넣어주고
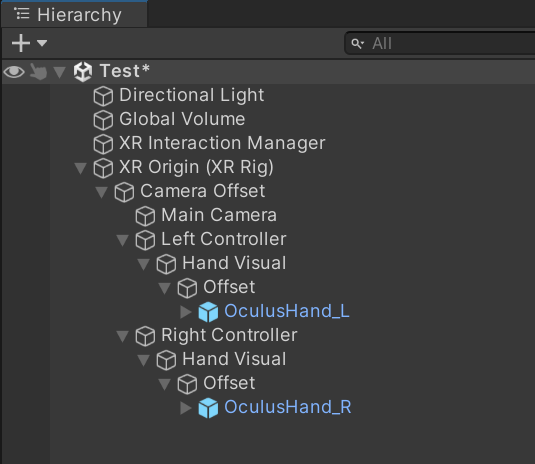
왼손 Offset 설정

오른손 Offset설정


using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.XR;
public class Hand : MonoBehaviour
{
[SerializeField] private InputDeviceCharacteristics inputDeviceCharacteristics;
[SerializeField] private Animator anim;
private InputDevice targetDevice;
void Start()
{
this.StartCoroutine(this.WaitForGetDevices());
}
private IEnumerator WaitForGetDevices()
{
WaitForEndOfFrame wait = new WaitForEndOfFrame();
List<InputDevice> devices = new List<InputDevice>();
while (devices.Count == 0)
{
yield return wait;
InputDevices.GetDevicesWithCharacteristics(inputDeviceCharacteristics, devices);
}
this.targetDevice = devices[0];
}
void Update()
{
if (this.targetDevice.isValid)
{
UpdateHand();
}
}
private void UpdateHand()
{
if (this.targetDevice.TryGetFeatureValue(CommonUsages.grip, out float gripValue))
{
this.anim.SetFloat("Grip", gripValue);
}
if (this.targetDevice.TryGetFeatureValue(CommonUsages.trigger, out float triggerValue))
{
this.anim.SetFloat("Trigger", triggerValue);
}
}
}
Grabbble 큐브 만들기

Grabbable Cube를 선택하고 rigidbody, xr grab interactable컴포넌트를 부착 한다
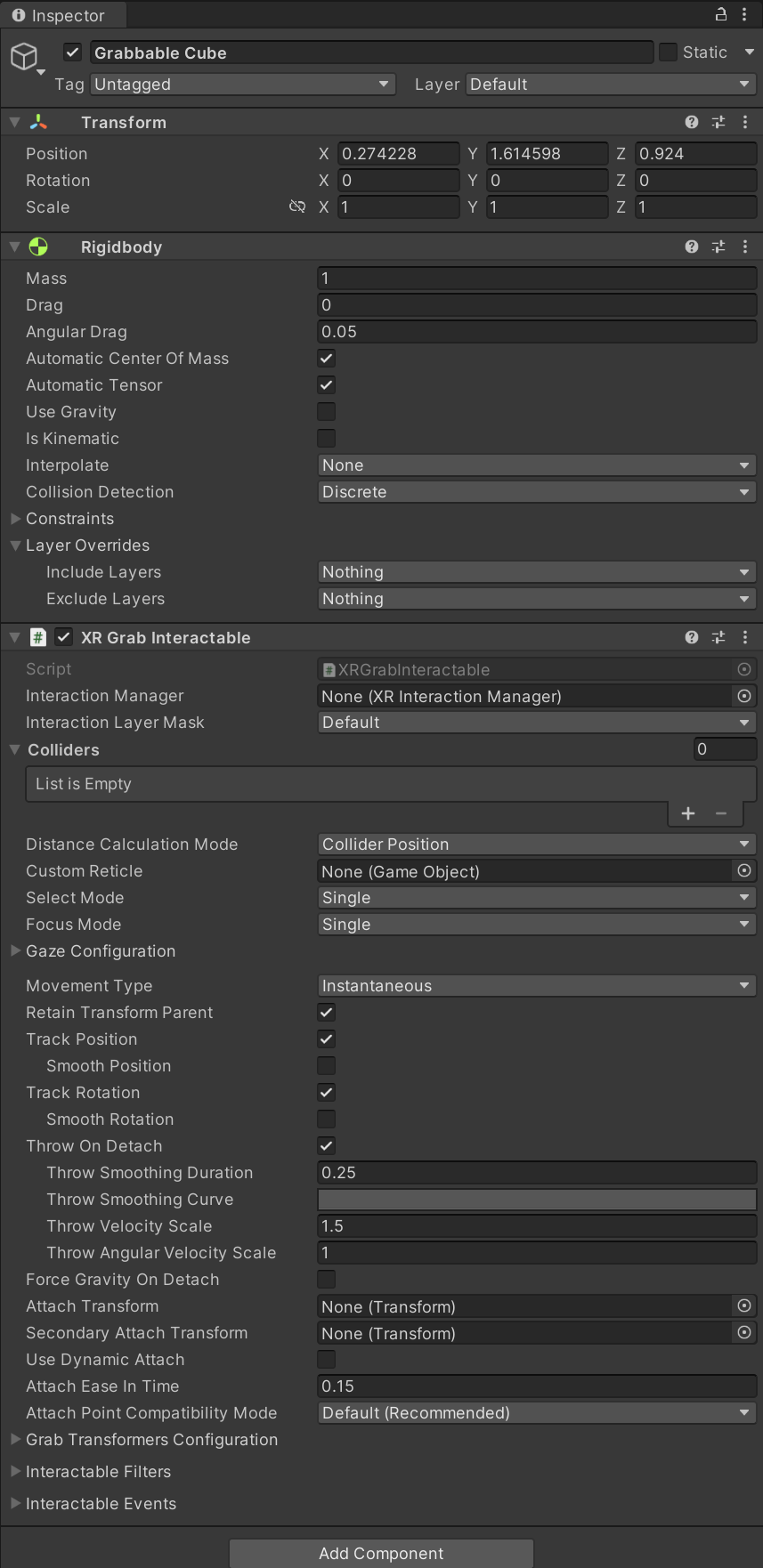
인터렉터 설정
프로젝트 창에서 Direct Interactor를 찾아 Left/Right Controller에 자식으로 넣어준다
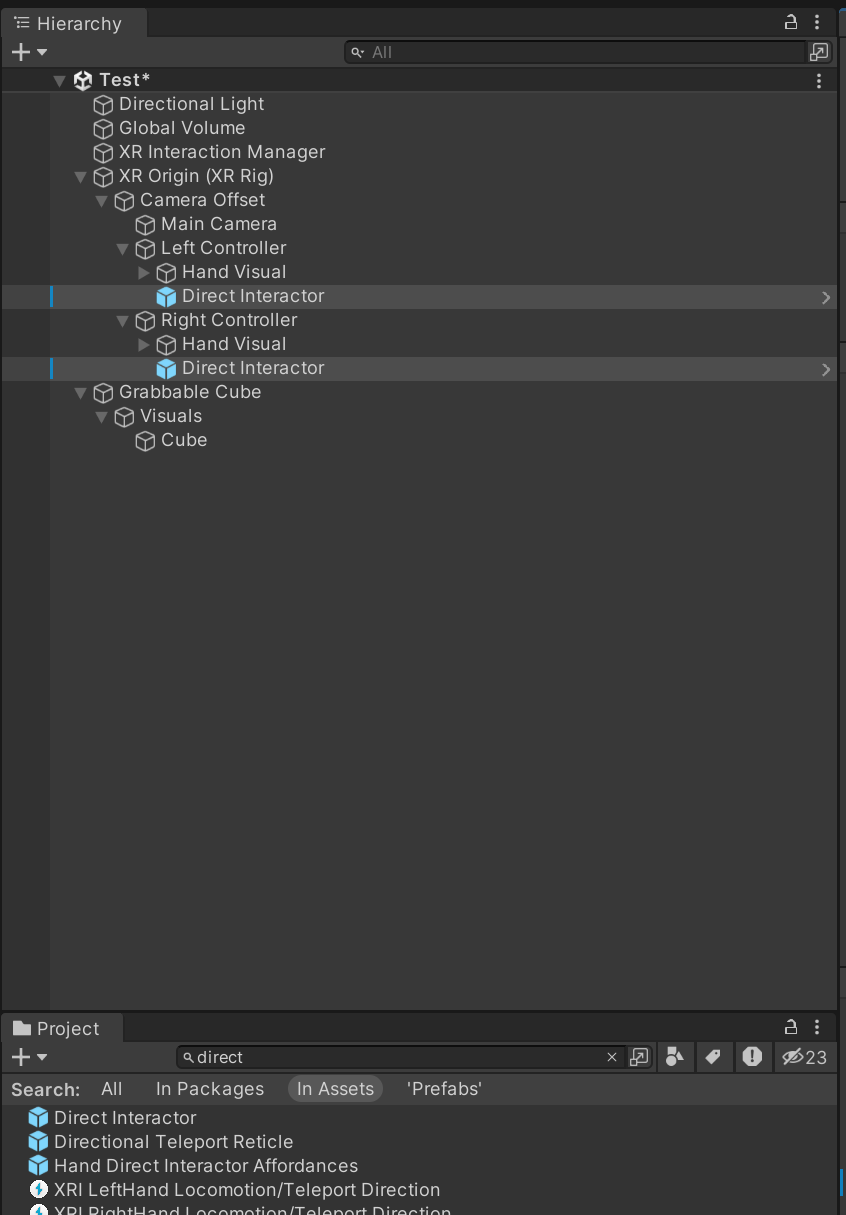
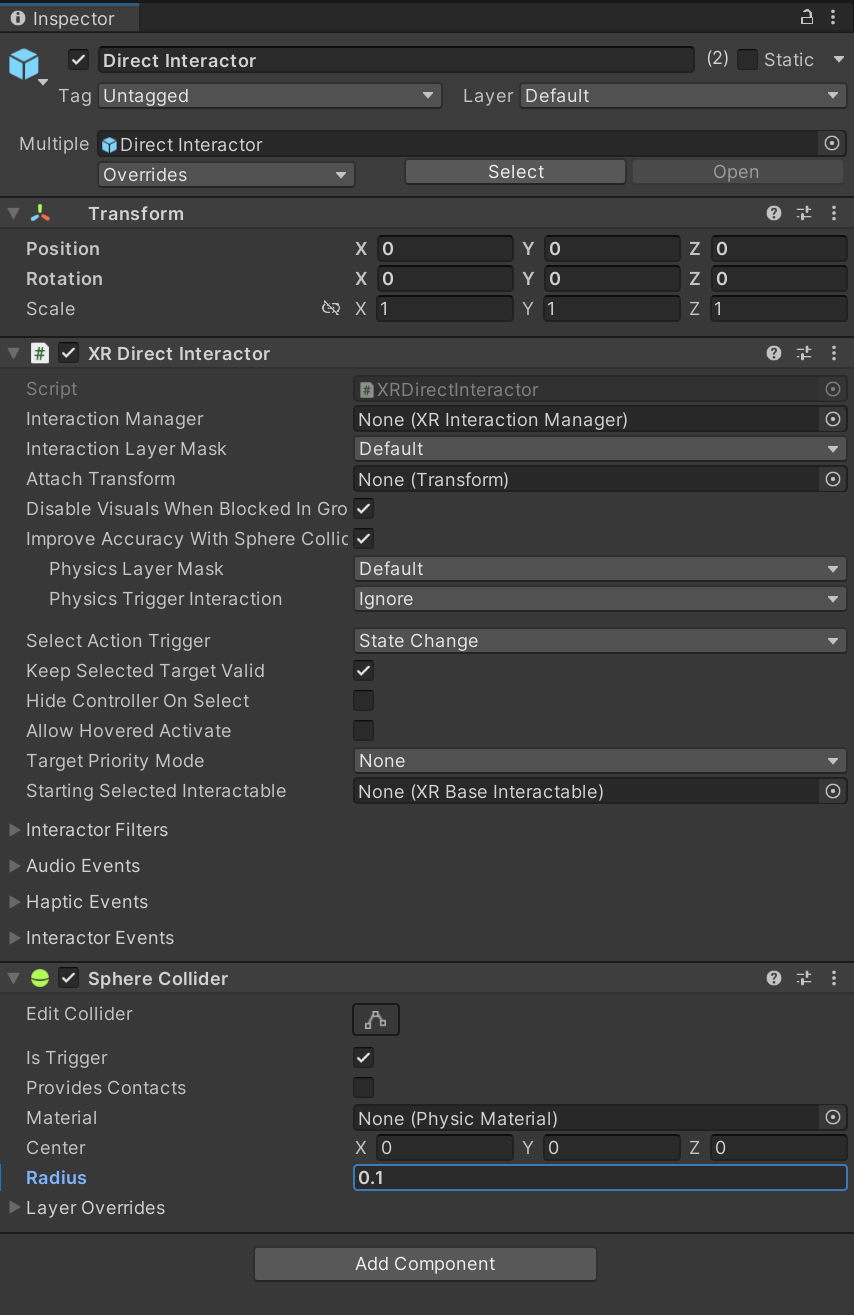
실행후 결과를 확인 한다
물체를 잡았다가 놓으면 고정되지 않고 떠다니는 현상이 발생하는 것은 Throw On Detach가 활성화 상태이기 때문이다

이 현상을 막기 위해서는 Throw On Detach를 비활성화 하거나 Rigidbody의 Is Kinematic을 체크 한다
실행하면 자동으로 생성 또는 할당되는 컴포넌트 확인하기
Left Controller의 Model 프로퍼티
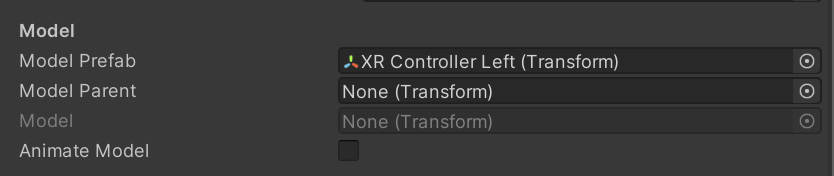

Grabbable Cube의 XR Grab Interactable 컴포넌트
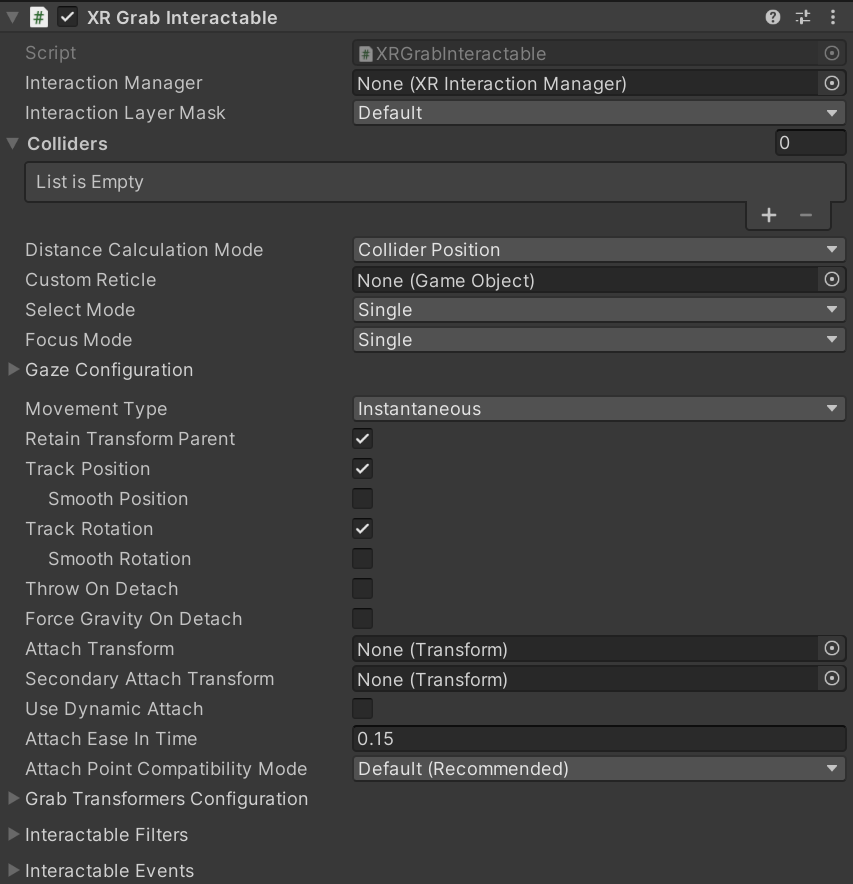
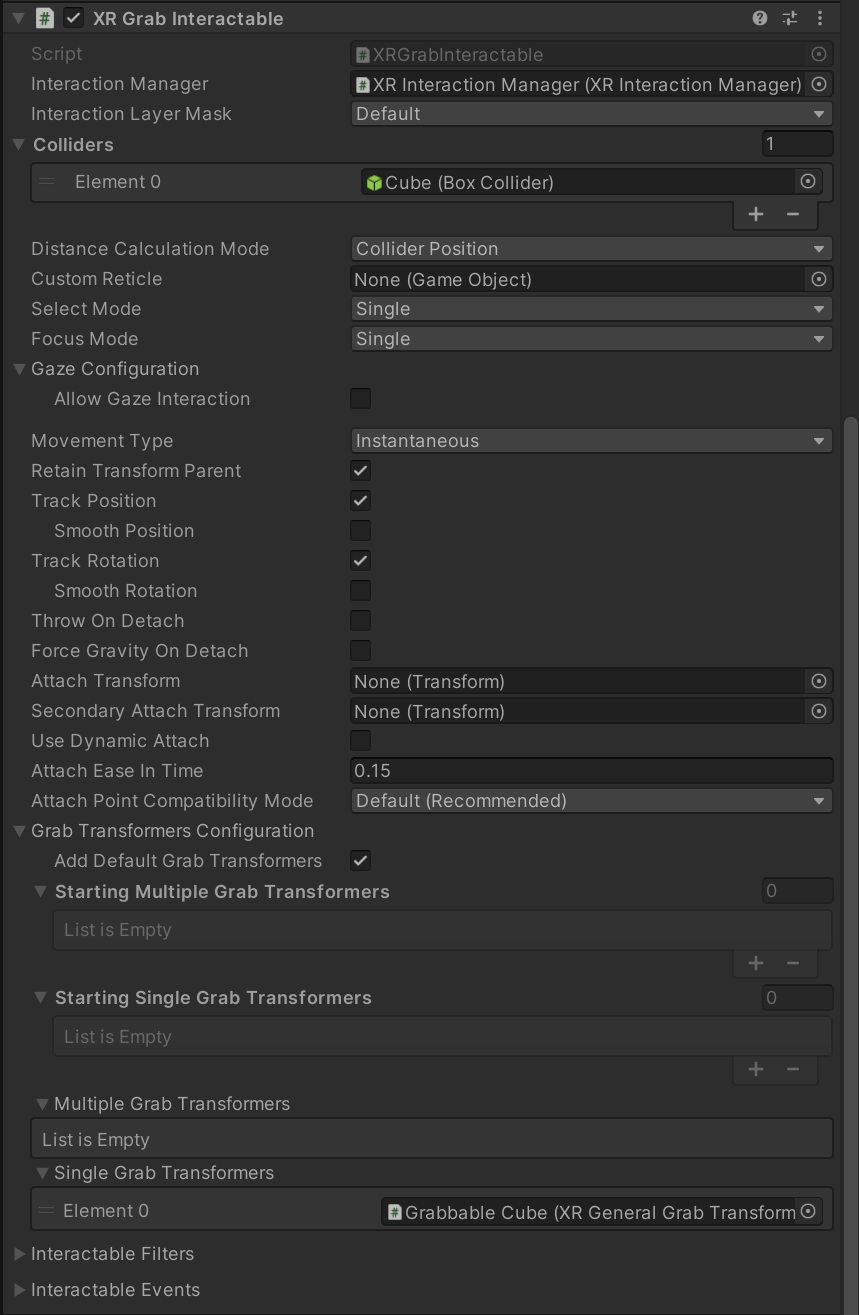
XR Grab Interactable컴포넌트가 부착된 Grabbable Cube에
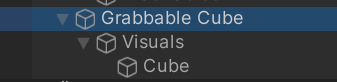
XR General Grab Transformer가 자동으로 부착 된다
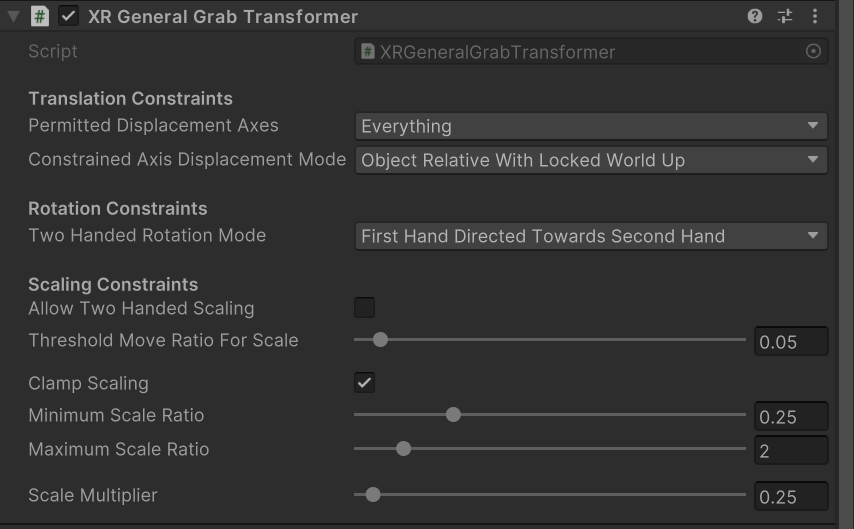
기본 Attach Point 확인 하기
박스의 중앙과 각 손의 손목에 색있는 구를 만들고 확인 해본다
정확히 손목으로 들어오는것을 확인 할수 있음
이제 문서를 확인 해보자
XR Grab Interactable
XR Grab Interactable | XR Interaction Toolkit | 2.4.3
XR Grab Interactable Interactable component that allows for basic grab functionality. When this behavior is selected (grabbed) by an Interactor, this behavior will follow it around and inherit velocity when released. Property Description Interaction Manage
docs.unity3d.com
기본 잡기 기능을 허용하는 상호 작용 가능한 구성 요소입니다. 이 동작이 인터랙터에 의해 선택(잡아)지면 이 동작은 이를 따라가며 놓을 때 속도를 상속합니다.
Interaction Manager | The XRInteractionManager that this Interactable will communicate with (will find one if None). |
없으면 찾아서 넣는다
Interaction Manager는 Interactor와 Interactable 사이의 중개자 역할을 합니다.
각각 고유한 유효한 인터랙터 및 인터랙터블 세트를 포함하는 여러 인터랙션 관리자를 가질 수 있습니다.
활성화되면 Interactor와 Interactable 모두 유효한 Interaction Manager에 등록됩니다(특정 관리자가 검사기에 아직 할당되지 않은 경우).
로드된 장면에는 Interactor 및 Interactable이 통신할 수 있도록 Interaction Manager가 하나 이상 있어야 합니다.
Interactors 및 Interactables의 많은 메서드는 상호 작용 이벤트의 두 대상 간의 일관성을 유지하기 위해 직접 호출되지 않고 이 Interaction Manager에 의해 호출되도록 설계되었습니다.
Interaction Layer Mask | Allows interaction with Interactors whose Interaction Layer Mask overlaps with any Layer in this Interaction Layer Mask. |
상호 작용 레이어 마스크가 이 상호 작용 레이어 마스크의 레이어와 겹치는 인터랙터와의 상호 작용을 허용합니다.
큐브를 하나 복사 하고
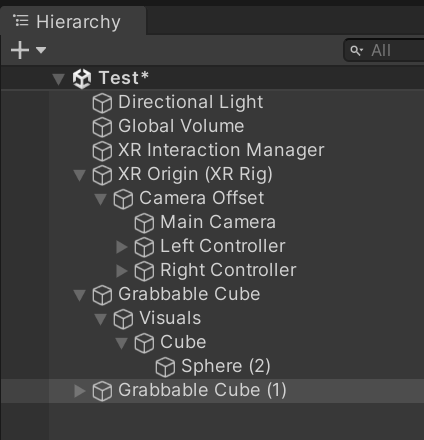
복사된 큐브를 선택하고
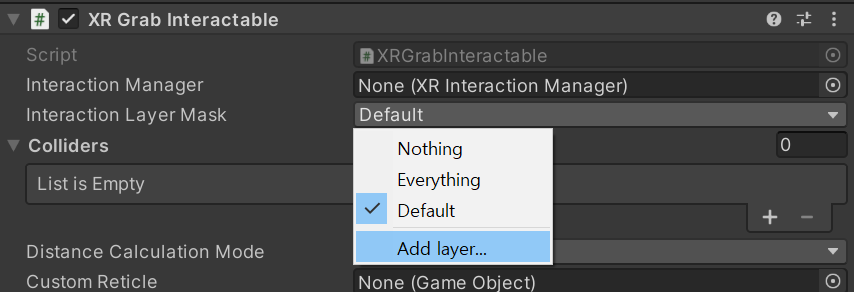
인터렉션 레이어를 추가 하고
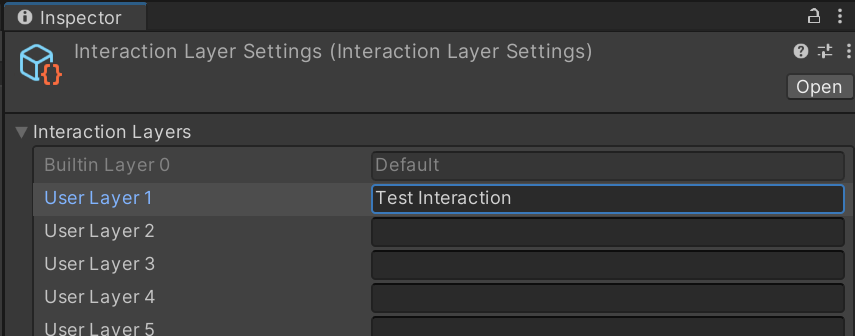
인터렉션 레이어 마스크를 변경한다

이제 왼손 컨트롤러의 Direct Interactor를 선택후
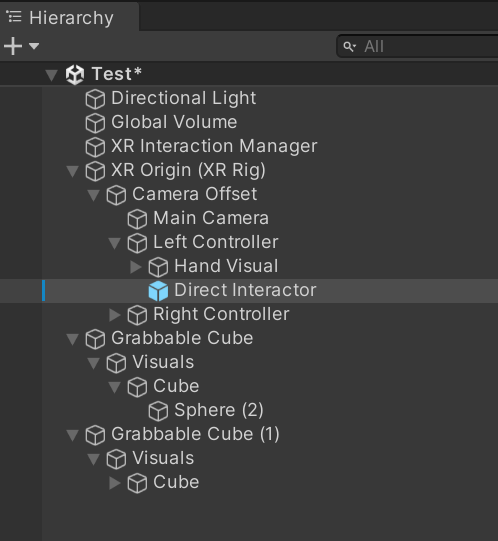
인터렉션 레이어 마스크를 설정 한다
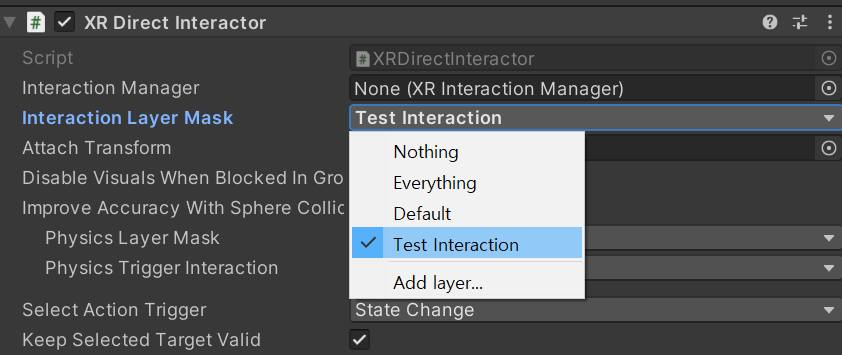
이 Interactable과의 상호 작용에 사용할 충돌체(비어 있는 경우 하위 충돌체를 사용함)
Colliders | Colliders to use for interaction with this Interactable (if empty, will use any child Colliders). |
다음과 같이 콜라이더를 하나 더 만들고 실행해 본다
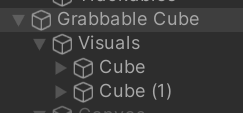
자동으로 하위 콜라이더들이 할당 되었다

가장 빠른 것부터 가장 정확한 것까지 인터랙터까지의 거리가 계산되는 방식을 지정합니다.
Mesh Colliders를 사용하는 경우 Collider Volume은 메쉬가 볼록한 경우에만 작동합니다.
Distance Calculation Mode | Specifies how distance is calculated to Interactors, from fastest to most accurate. If using Mesh Colliders, Collider Volume only works if the mesh is convex. |
Unity의 XR Interaction Toolkit에서 XR Grab Interactable 컴포넌트의 Distance Calculation Mode 속성은 인터랙터와 상호작용 가능한 객체 간의 거리를 계산하는 방법을 제공합니다. 이 옵션들을 이해하는 것은 XR 애플리케이션의 성능과 사용자 경험을 최적화하는 데 중요합니다. 각 모드에 대한 자세한 설명은 다음과 같습니다:
- Transform Position (변환 위치):
- 설명: 이 모드는 상호작용 가능한 객체의 변환 위치를 사용하여 거리를 계산합니다.
- 성능 비용: 낮음.
- 거리 계산 정확도: 일부 객체에 대해 정확도가 낮을 수 있습니다. 이는 객체의 중심점을 기준으로 거리를 계산하기 때문에, 크기가 크거나 복잡한 형태의 객체에서는 정확도가 떨어질 수 있습니다.
- Collider Position (콜라이더 위치):
- 설명: 이 모드는 상호작용 가능한 객체의 콜라이더 목록을 사용하여 각 콜라이더까지의 가장 짧은 거리를 계산합니다.
- 성능 비용: 중간.
- 거리 계산 정확도: 대부분의 객체에 대해 중간 정도의 정확도를 제공합니다. 이 방법은 콜라이더의 위치를 기반으로 거리를 계산하기 때문에, 객체의 형태가 복잡하거나 여러 콜라이더를 가진 경우에도 적절한 정확도를 제공할 수 있습니다.
- Collider Volume (콜라이더 볼륨):
- 설명: 이 모드는 상호작용 가능한 객체의 콜라이더 목록을 사용하여 각 콜라이더의 가장 가까운 지점까지의 거리를 계산합니다(콜라이더의 표면이나 내부에 있는 지점 포함).
- 성능 비용: 높음.
- 거리 계산 정확도: 높음. 이 방법은 콜라이더의 볼륨 내부나 표면에 있는 가장 가까운 지점까지의 거리를 계산하기 때문에, 가장 정확한 거리 측정을 제공하지만 성능 비용이 높습니다.
각 모드는 성능과 정확도의 균형을 고려하여 선택해야 합니다. 애플리케이션의 요구 사항과 사용자 경험에 따라 적절한 모드를 선택하는 것이 중요합니다.
Transform Position | Interactable의 변환 위치를 사용하여 거리를 계산합니다. 이 옵션은 성능 비용이 낮지만 일부 객체의 경우 거리 계산 정확도가 낮을 수 있습니다. |
Collider Position | Interactable의 Colliders 목록을 사용하여 각각에 대한 최단 거리를 사용하여 거리를 계산합니다. 이 옵션은 성능 비용이 적당하며 대부분의 객체에 대해 적당한 거리 계산 정확도를 가져야 합니다. |
Collider Volume | Interactable의 Colliders 목록을 사용하여 각각의 가장 가까운 지점(표면 또는 Collider 내부)까지의 최단 거리를 사용하여 거리를 계산합니다. 이 옵션은 성능 비용이 높지만 거리 계산 정확도가 높습니다. |
Custom Reticle | The reticle that appears at the end of the line when valid. |

Left Controller자식으로 Ray Interactor 프리팹을 넣는다
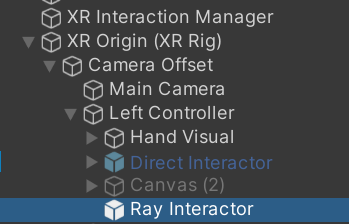
레티클용 프리팹을 만들어주고
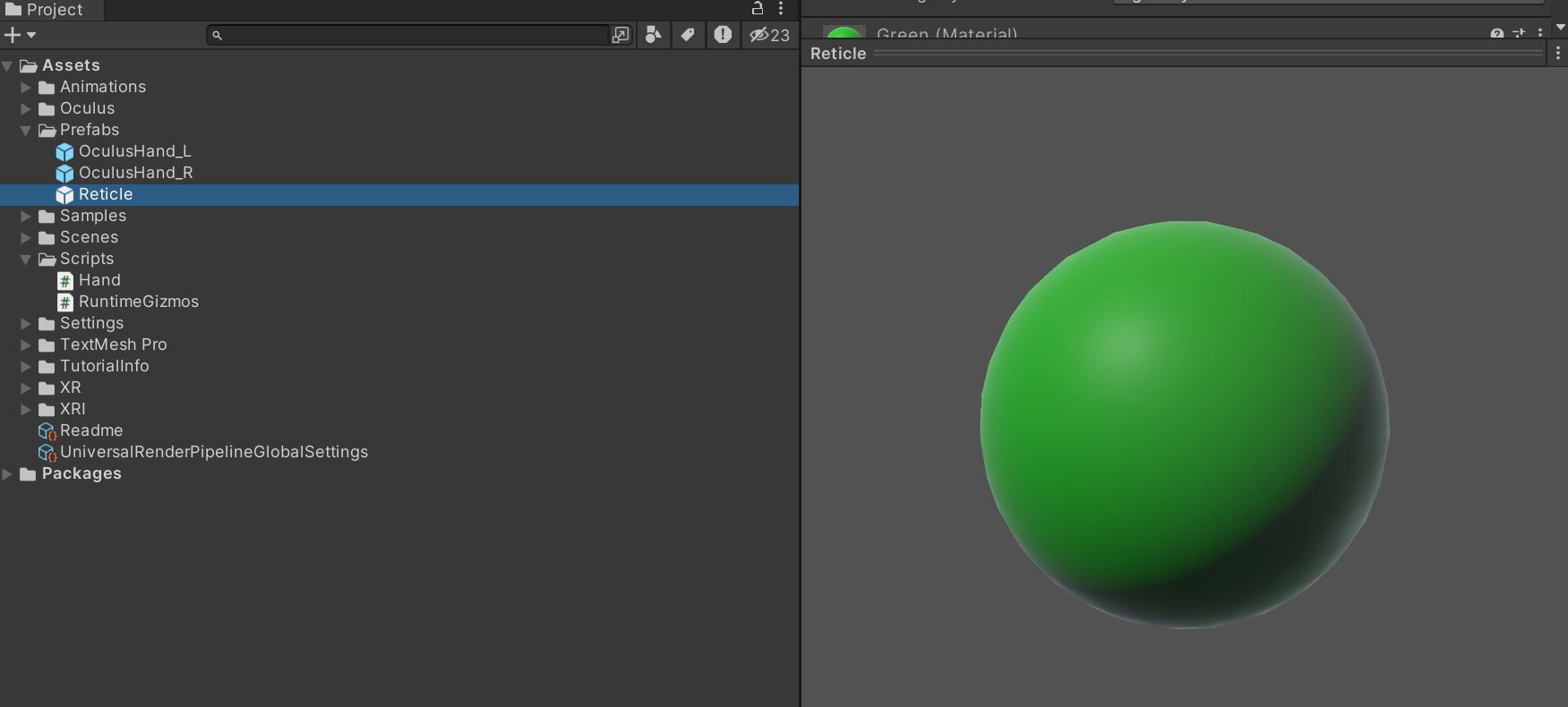
Custom Reticle 프로퍼티에 넣어준다

Focus Mode | Specifies the focus policy of this interactable. |
None | Set Focus Mode to None to disable the foucs state for an interactable. |
Single | Set Focus Mode to Single to allow interactors of a single interaction group to focus this interactable. |
Multiple | Set Focus Mode to Multiple to allow interactors of multiple interaction groups to focus this interactable |
Architecture | XR Interaction Toolkit | 2.4.3
Architecture This section describes the relationship between the core components of the interaction system and the states that make up the lifecycle of an interaction. States The Interaction system has three common states: Hover, Select, and Activate. Thes
docs.unity3d.com
Architecture | XR Interaction Toolkit | 2.4.3
Architecture This section describes the relationship between the core components of the interaction system and the states that make up the lifecycle of an interaction. States The Interaction system has three common states: Hover, Select, and Activate. Thes
docs.unity3d.com